(Cancelled) shuttle.rs Christmas Code Hunt
Shuttle Christmas Code Hunt: An early start to a month of puzzling.
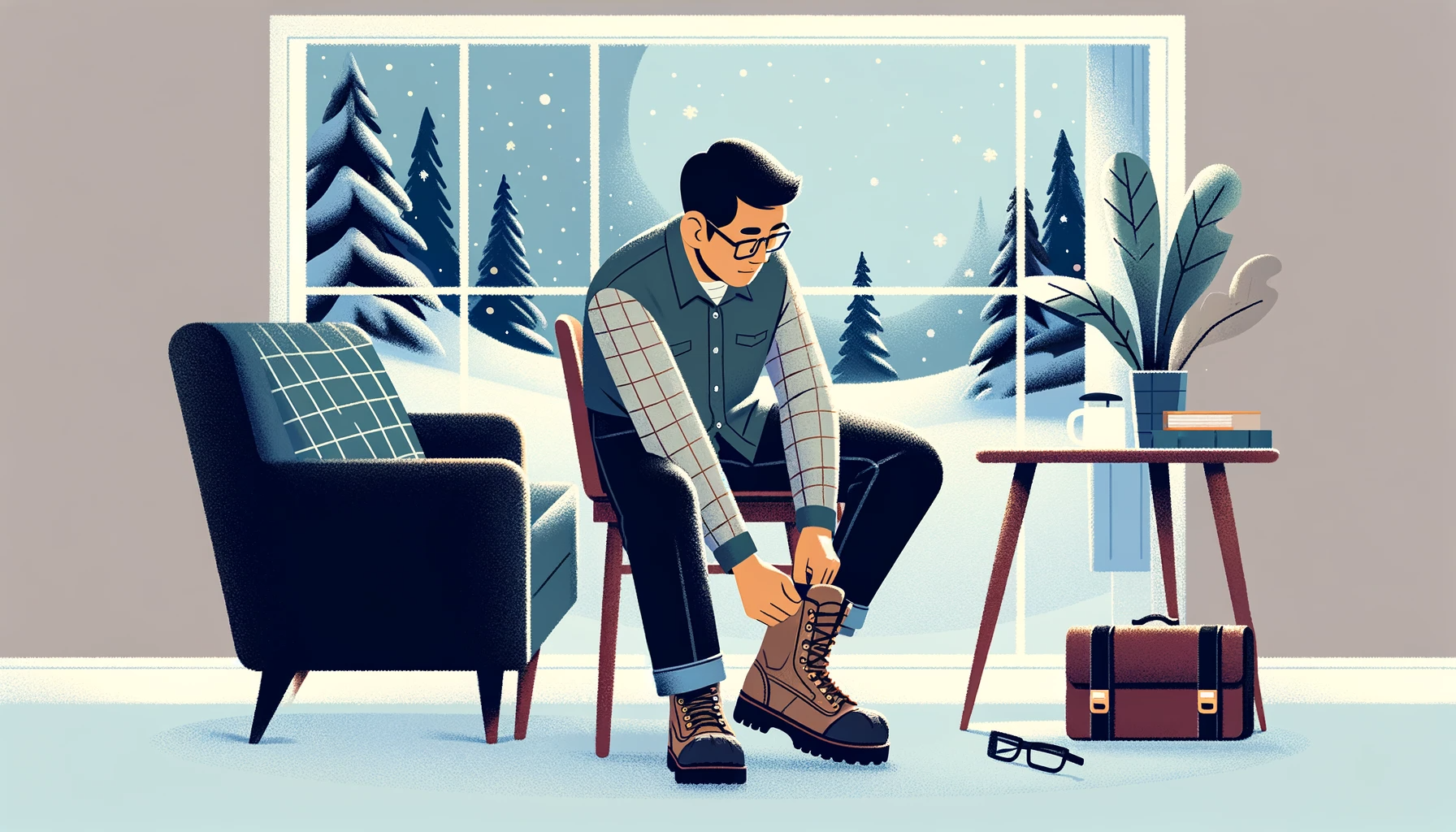
Shuttle.rs is putting on an advent of code, and I want an excuse to learn some back-end programming and some Rust. So, lets get to learning. This is going to mostly be me fumbling through Rust and some web frameworks, but I'll also give some honest feedback about shuttle.rs as well.
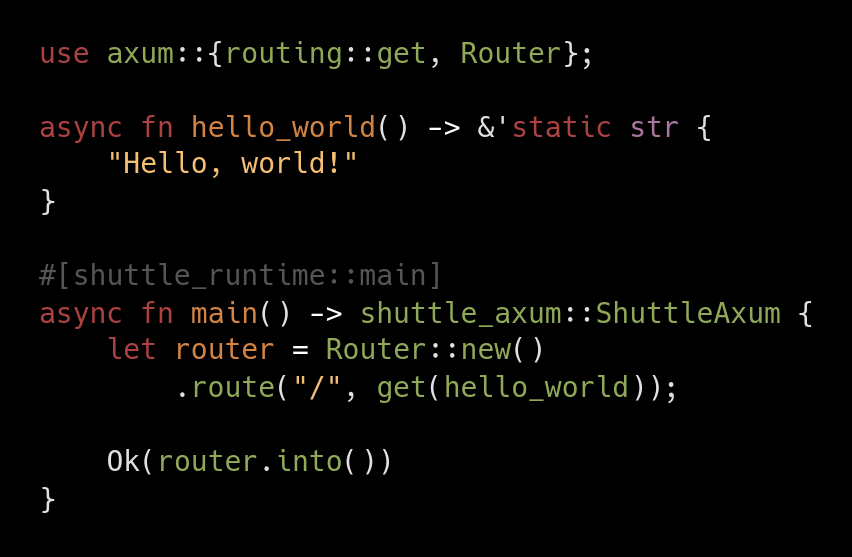
Day -1: Get your winter boots on!
Today was just about getting set up with the service. I think this was a necessary introduction since it establishes usage of their site. Setup is a breeze, the hardest part by far is deciding which web framework to use. I later found that they have a detailed breakdown of the supported frameworks, but after doing my own research I went with Rocket. Code snippets of Rocket made the most sense to me, I understand there are some negatives to their approach specifically around macros, but I'll happily make that trade for ease of use.
Task 1
The challenge today was solved by the default Rocket template.
use rocket::{get, routes};
use rocket::http::Status;
#[get("/")]
fn index() -> &'static str {
"Hello, world!"
}
#[shuttle_runtime::main]
async fn main() -> shuttle_rocket::ShuttleRocket {
let rocket = rocket::build().mount("/", routes![index, error]);
Ok(rocket.into())
}
No changes necessary 😎
Task 2
Just like Advent of Code there is a stretch goal for an additional internet point. They want the route "/-1/error"
to return a 501 Internal Server Error
. A small addition to the code:
use rocket::{get, routes};
use rocket::http::Status;
#[get("/")]
fn index() -> &'static str {
"Hello, world!"
}
#[get("/-1/error")]
fn error() -> Status {
Status::InternalServerError
}
#[shuttle_runtime::main]
async fn main() -> shuttle_rocket::ShuttleRocket {
let rocket = rocket::build().mount("/", routes![index, error]);
Ok(rocket.into())
}
and bam that is another internet point in the bag 😎
Additional Initial Setup
This code is going to also live on GitLab, so I also gave the project an MIT license and some simple CI stuff to keep me on my toes:
stages:
- test
- build
image: rust:latest
before_script:
- rustup component add clippy
- rustup component add rustfmt
clippy:
stage: test
script:
- cargo clippy -- -D warnings
rustfmt:
stage: test
script:
- cargo fmt -- --check
build:
stage: build
script:
- cargo build --verbose
Check out the repo here (likely to be filled with spoilers soon), and hopefully this series makes it to Christmas 🎄
Day 0.1: The Woes of Getting Users
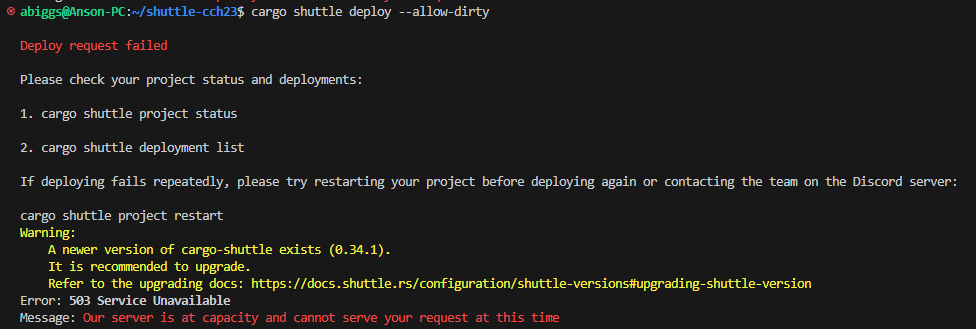
Shuttle did not survive first contact with the enemy. Once the code hunt commenced and users flooded their servers with solutions shuttle.rs ground to a halt. They haven't posted any metrics on their Discord but its safe to say their service usage probably saw some orders of magnitude increase in usage overnight.
In the moment this is horrifying, but in retrospect they've managed to seriously stress test their service for free, while (hopefully) causing minimal disruption to their paying customers. The communication has been kind of lacking, and I do worry a lot that their status page hasn't show a single blip during this whole saga. The team says to only expect communication through Discord, which I think is fine for a small community or an open-source project, but if I'm paying for a SaaS I expect more. Hopefully they put up a post-mortem at some point.
As of December 4th the service is still shut down for the Christmas Code Hunt.
They eventually cancelled the code hunt. The puzzles are still available but there is no integration with their service at all and they released code to run the tests locally instead of on shuttle. This kind of killed the point for me since I wanted to use this to learn the platform so as of now I'm not finishing this series.